我们想让异常结果也显示为统一的返回结果对象,并且统一处理系统的异常信息,那么需要统一异常处理。
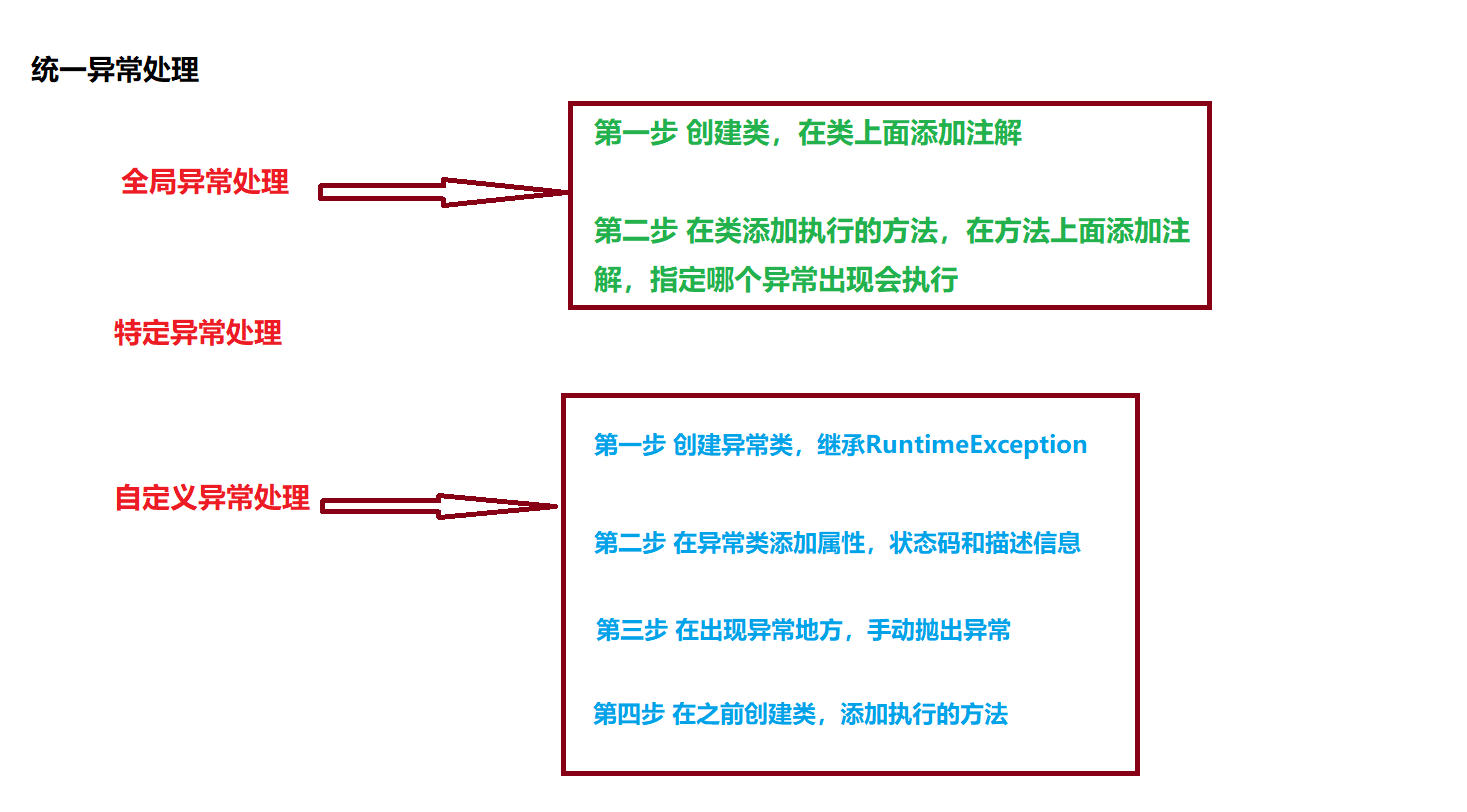
1.全局异常处理
异常返回的结果也为统一的返回结果的对象
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49
| package com.atguigu.common.exception;
import com.atguigu.common.result.Result; import org.springframework.web.bind.annotation.ControllerAdvice; import org.springframework.web.bind.annotation.ExceptionHandler; import org.springframework.web.bind.annotation.ResponseBody;
@ControllerAdvice public class GlobalExceptionHandler {
@ExceptionHandler(Exception.class) @ResponseBody public Result error(Exception e){ e.printStackTrace(); return Result.fail().message("全局异常处理器捕获到该异常"); }
@ExceptionHandler(ArithmeticException.class) @ResponseBody public Result error(ArithmeticException e){ e.printStackTrace(); return Result.fail().message("数学运算异常"); }
@ExceptionHandler(GuiguException.class) @ResponseBody public Result error(GuiguException e){ e.printStackTrace(); return Result.fail().code(e.getCode()).message(e.getMessage()); } }
|
2.自定义异常
创建异常类 继承RuntimeException
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53
| package com.atguigu.common.exception;
import com.atguigu.common.result.ResultCodeEnum; import lombok.Data;
@Data public class GuiguException extends RuntimeException {
private Integer code;
private String message;
public GuiguException(Integer code, String message) { super(message); this.code = code; this.message = message; }
public GuiguException(ResultCodeEnum resultCodeEnum) { super(resultCodeEnum.getMessage()); this.code = resultCodeEnum.getCode(); this.message = resultCodeEnum.getMessage(); }
@Override public String toString() { return "GuliException{" + "code=" + code + ", message=" + this.getMessage() + '}'; }
}
|
在需要抛出自定义异常的地方抛出异常
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15
|
@ApiOperation("查询所有角色的接口") @GetMapping("/findAll") public Result<List<SysRole>> findAll() { List<SysRole> sysRoles = sysRoleService.list(); try { int i = 1/0; } catch (Exception e) { throw new GuiguException(2001, "抛出了自定义的异常"); } return Result.ok(sysRoles); }
|
全局异常处理器捕获异常并返回异常信息
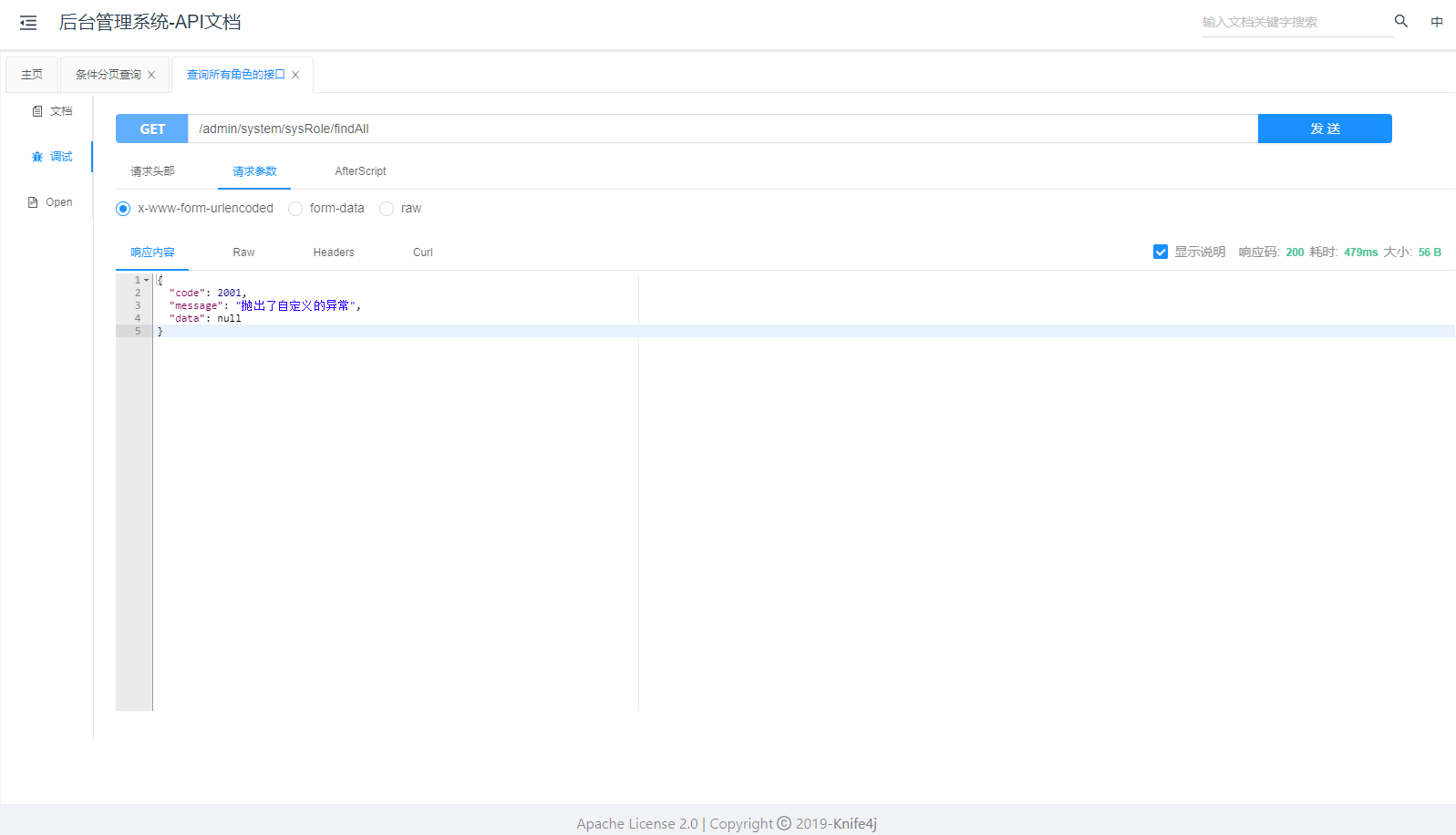